How to Initialize Multiple Variables in Java
- Java Howtos
- How to Initialize Multiple Variables in …
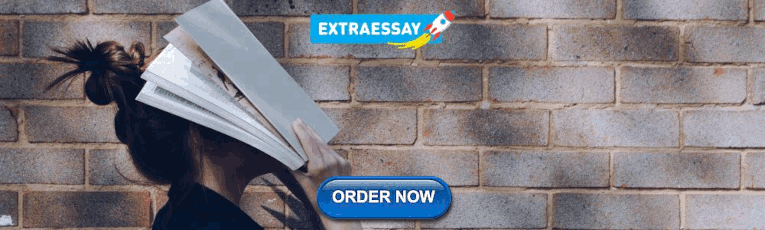
Importance of Initializing Multiple Variables with the Same Value
Initialize multiple string variables with the same value in java.
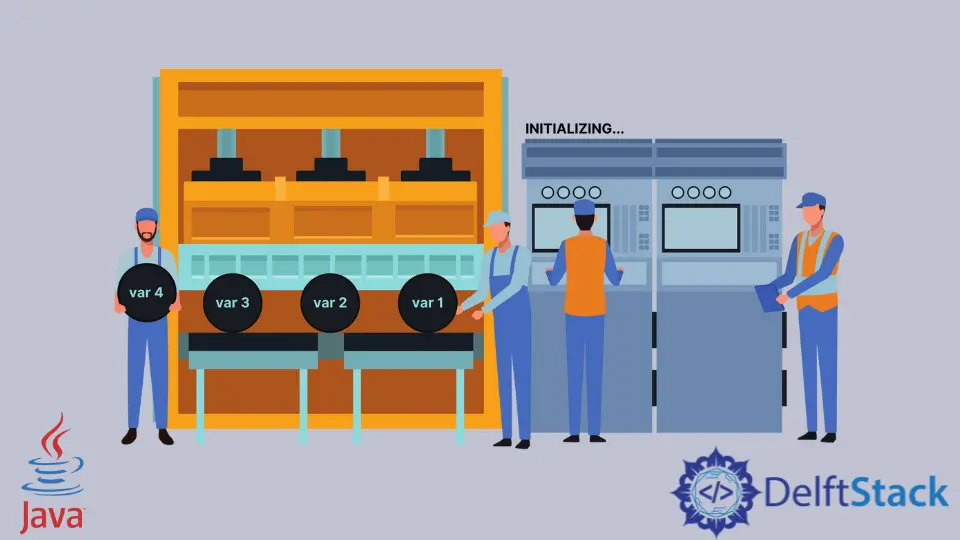
This article delves into the significance of initializing multiple variables with the same value and explores various methods to achieve this essential programming task.
Initializing multiple variables with the same value is essential for maintaining consistency and ensuring uniformity in a program. It simplifies code maintenance, reduces errors, and enhances readability.
When related variables share a common initial value, it fosters clarity in understanding code logic and promotes efficient use of resources. This practice is particularly crucial in scenarios where a group of variables should start with identical values, ensuring cohesive and predictable behavior in the program.
Inline Declaration and Initialization
The inline declaration and initialization method is crucial for concise code. Combining variable declaration and assignment in a single line minimizes redundancy and enhances readability.
This approach is efficient and effective, reducing the need for multiple lines and making it advantageous over alternative methods for initializing multiple variables with the same value.
Let’s consider a scenario where we need to initialize three integer variables ( a , b , and c ) with the same value (let’s say 10 ). Instead of repetitive individual assignments, we can leverage inline declaration and initialization to achieve this concisely.
In this code breakdown, we examine the significance of the inline declaration and initialization method.
Initially, three integer variables ( a , b , and c ) are declared and initialized in a single line, merging the variable type ( int ) with the assignment. This streamlined approach reduces code verbosity.
Subsequently, we display the initialized values of a , b , and c on the console, ensuring the successful implementation of the inline declaration and initialization method. This method proves crucial for maintaining code conciseness and verifying accurate variable initialization.
By employing inline declaration and initialization, we achieve a concise and expressive way of initializing multiple variables with the same value. The output of the provided code will be as follows:
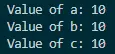
This output confirms that all three variables ( a , b , and c ) have been initialized with the desired value ( 10 ). Utilizing inline declaration and initialization not only enhances code readability but also promotes a more efficient and elegant coding style, making your Java programs more maintainable and less prone to errors.
Chained Assignments Method
The chained assignments method is pivotal for concise and readable code. It reduces redundancy by assigning values in a sequential manner, enhancing the efficiency of variable initialization.
This approach streamlines the process, making the code more compact and maintaining a clear logical flow, providing a distinct advantage over alternative methods.
Consider a scenario where three integer variables ( a , b , and c ) need to be initialized with the same value, let’s say 15 . The chained assignments method provides a concise and expressive way to achieve this:
Let’s analyze the code to comprehend the intricacies of the chained assignments method.
In the initial step, we declare and assign values to three integer variables ( a , b , and c ) in a single line, employing chained assignments. The process flows from right to left, with the rightmost value ( 15 ) assigned to c , followed by the value of c assigned to b , and ultimately, the value of b assigned to a .
Subsequently, we display the initialized values of a , b , and c on the console, ensuring the successful implementation of the chained assignments method for uniform initialization.
By employing the chained assignments method, we achieve a concise and expressive way of initializing multiple variables with the same value. The output of the provided code will be as follows:
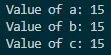
This output confirms that all three variables ( a , b , and c ) have been initialized with the desired value ( 15 ). The chained assignments method offers an elegant solution to streamline code while maintaining clarity, making it a valuable technique for enhancing the readability and efficiency of your Java programs.
Common Value Method
Initializing multiple variables with the same value using the common value method reduces redundancy and promotes code clarity by providing a single point of reference for initialization. This approach simplifies maintenance and ensures consistency, making code more concise and readable compared to other methods.
Consider a scenario where three integer variables ( a , b , and c ) need to be initialized with the same value, say 30 . The common value method provides a clean and concise solution:
In the code example, we employ the common value method to initialize multiple variables with the same value.
Initially, a variable named commonValue is declared and assigned the desired value ( 30 ). This single point of reference is then utilized to initialize three integer variables ( a , b , and c ).
By doing so, we minimize redundancy and enhance code clarity. The initialized values of these variables are subsequently displayed on the console.
This approach, known as the common value method, proves to be a simple and readable technique for achieving uniform initialization in Java.
By employing the common value method, we achieve a simple and effective way of initializing multiple variables with the same value. The output of the provided code will be as follows:
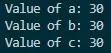
This output confirms that all three variables ( a , b , and c ) have been successfully initialized with the desired value ( 30 ). The common value method is particularly beneficial when dealing with a small number of variables, offering a clear and concise solution to the task of variable initialization in Java.
Array Method
The array method for initializing multiple variables with the same value is crucial for structured organization and uniformity. It minimizes redundancy, streamlines code, and provides a clear container for related variables.
This approach simplifies maintenance and promotes a concise, readable code structure, making it advantageous over other methods.
Imagine a scenario where you need to initialize three integer variables ( a , b , and c ) with the same value, say 20 . The array method provides an organized and efficient solution:
In the code, the array method for initializing multiple variables involves several essential steps.
Initially, an array of integers named variables is declared, providing a container for the related variables with a length of 3 . Following this, a variable named commonValue is declared and set to the desired shared value.
The core of the method lies in a for loop, where each element of the variables array is iterated, and the commonValue is assigned, achieving consistent initialization.
The code concludes by displaying the initialized values of the variables ( a , b , and c ). This method offers a structured approach, enhancing code organization and maintaining uniformity.
By employing the array method, we achieve an organized and efficient way of initializing multiple variables with the same value. The output of the provided code will be as follows:
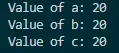
This output confirms that all three variables ( a , b , and c ) have been successfully initialized with the desired value ( 20 ). The array method provides a structured approach to handling related variables, contributing to cleaner and more maintainable Java code.
In conclusion, understanding the significance of initializing multiple variables with the same value is fundamental for code consistency and readability. This article has explored various effective methods, including inline declaration, chained assignments, common value, and array usage.
Choosing the right approach depends on the specific context of the program. By embracing these techniques, developers can streamline their code, reduce redundancy, and ensure a cohesive and predictable initialization of variables, contributing to the overall clarity and maintainability of Java programs.
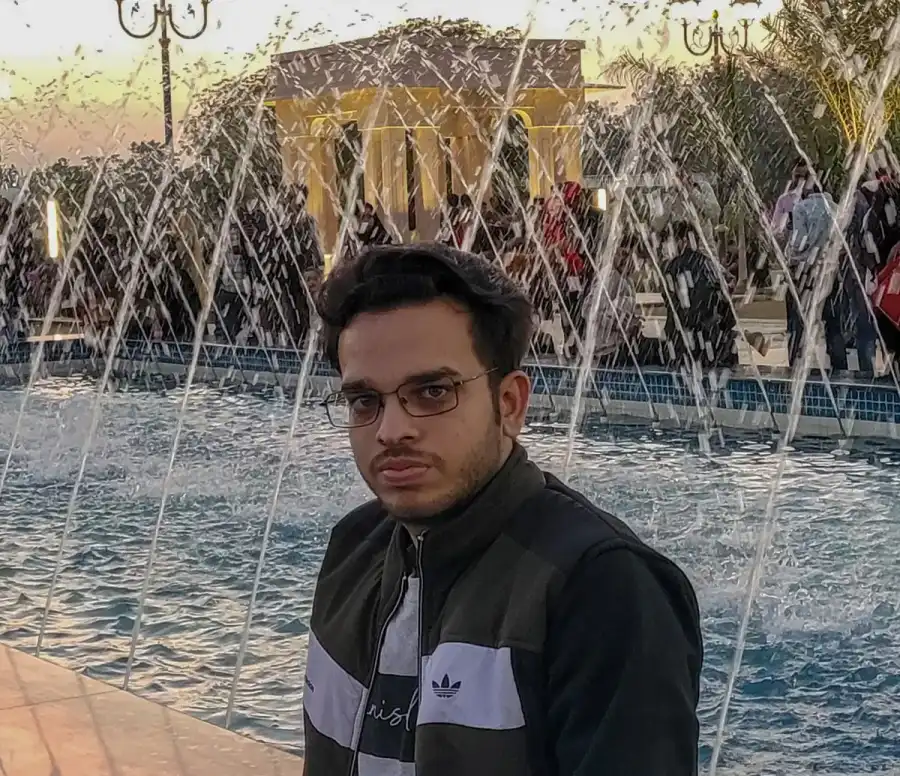
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
Related Article - Java Variable
- Java Synchronised Variable
- Difference Between Static and Final Variables in Java
- How to Set JAVA_HOME Variable in Java
- How to Create Counters in Java
- How to Increase an Array Size in Java
- How to Access a Variable From Another Class in Java

Get Started: Java Declare Multiple Variables
- August 23, 2022
In This Article, You Will Learn about Java Declare Multiple Variables.
Java Declare Multiple Variables – Before moving ahead, let’s know a bit about Java Variables .
Table of Contents
Declare many variables.
To declare more than one variable of same type, Java allows us to use comma-seprated list.
Example: Declare multiple variables of same type.
Alternatively;
One Value To Multiple Variables
Java allows us to assign a same value to the multiple variables in a same line.
Example: Declare same value to multiple variables.
If you find anything incorrect in the above-discussed topic and have further questions, please comment below.
Connect on:
Recent Post

Python Conditional Statements (if else) Project for Beginners

Google Dialogflow Chatbot – Renew Subscription Plan

How to Create a Google Dialogflow Chatbot – Solved Hosting Login Issues?

How to create a Chatbot with Google Dialogflow?

Google Dialogflow Chatbot Tutorial for Beginner
Popular post.

Network Security: Introduction to Network Security

Get Started: SQL Working With Dates

Introduction to Machine Learning Standard Deviation

Assumption of Linear Regression in Machine Learning

Network Security: Data Encryption Standard

Top Articles

Top 8 Reasons Why You Should Think of Python in 2022

Network Security: Different Types of Services and Mechanisms

What are the Different Types of Cloud Services?

Journey of DL & ML: Deep Learning vs Machine Learning

Python Program: Swap Two Values in Python
The output of this program is 57 , because the first time we print fred his value is 5, and the second time his value is 7.
This kind of multiple assignment is the reason I described variables as a container for values. When you assign a value to a variable, you change the contents of the container, as shown in the figure:
When there are multiple assignments to a variable, it is especially important to distinguish between an assignment statement and a statement of equality. Because Java uses the = symbol for assignment, it is tempting to interpret a statement like a = b as a statement of equality. It is not!
First of all, equality is commutative, and assignment is not. For example, in mathematics if a = 7 then 7 = a . But in Java a = 7; is a legal assignment statement, and 7 = a; is not.
Furthermore, in mathematics, a statement of equality is true for all time. If a = b now, then a will always equal b . In Java, an assignment statement can make two variables equal, but they don't have to stay that way!
The third line changes the value of a but it does not change the value of b , and so they are no longer equal. In many programming languages an alternate symbol is used for assignment, such as <- or := , in order to avoid this confusion.
Although multiple assignment is frequently useful, you should use it with caution. If the values of variables are changing constantly in different parts of the program, it can make the code difficult to read and debug.

Declaring Multiple Variables in Java
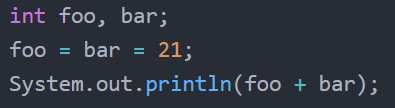
In Java , we can use a comma-separated list to simultaneously declare multiple variables of the same type. This can make it easy to declare sets of variables, reduces the size of our code and simplifies it.
Declaring Multiple Values Without Initializing
We can declare multiple variables of the same type on a single line without initializing them . The following example demonstrates this:
In this example, we have declared two integer variables foo and bar . We’ve left them uninitialized for now, but we will need to do so at some point before attempting to call them.
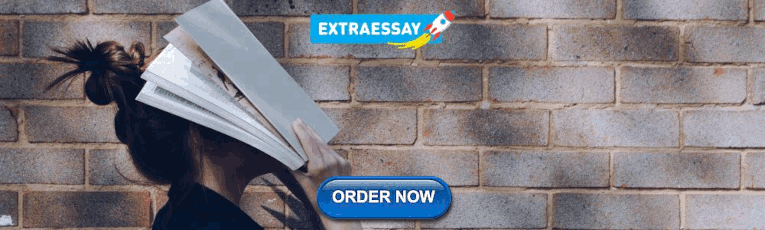
Declaring and Initializing Multiple Variables
We can also declare and initialize variables of the same type on a single line using the assignment ‘=’ operator:
Output : 42
In this case, we’ve successfully initialized foo to a value of 12 and bar to a value of 30. We then printed the sum of foo and bar to the console using System.out.println() .
Declare and Initialize Multiple Variables to the Same Value
If we have multiple variables of the same type that need to be initialized to the same value, we can declare them all on one line and then initialize them all on a separate line:
- Course List
- First Steps
- Introduction to Java
- Environment setup
- Workspace & First app
- Basic Syntax
- Variables & Constants
- Control Flow
- if, else-if, else, switch
- for, foreach, while, do-while loops
- Intermediate
- Object-oriented Programming
- OOP: Classes & Objects
- OOP: Methods
- OOP: Inheritance
- OOP: Composition
- OOP: Polymorphism
- OOP: Encapsulation
- Lambda Expressions
- Enums (Enumerations)
- List Collections
- ArrayList Collection
- Map Collections
- HashMap Collection
- Errors & Exceptions
- Error & Exception Handling
- Concurrency & Multi-threading
Java Variables & Constants Tutorial
In this Java tutorial we learn about variables and constants and how they store temporary data while the program runs.
We discuss how to declare or initialize variables and constants, how to use them and change variable values.
Finally, we look at operands in Java, lvalues and rvalues.
- What is a variable
Variable types in Java
How to declare a variable in java, how to assign a value to a variable in java, how to initialize a variable with a value in java, how to declare/initialize multiple variables inline in java, how to use a variable in java, how to change a variable's value in java.
- What is a constant
How to initialize a constant in Java
Java operands: lvalues and rvalues, examples of common variable data types in java.
- Summary: Points to remember
A variable is a temporary data container that stores a value in memory while the application is running.
As an example, let’s consider a calculator program.
When we perform an addition for example, the app stores the result in a variable that we can use to perform any additional calculations we need.
In the example above, the letters ‘x’ and ‘y’ store the resulting data from the arithmetic.
Variables are used all over our apps to store runtime data. When the app is closed, or the variable is no longer used by the program, the value in memory is removed.
There are three types of variables in java:
1. A local variable is defined within a function, or method. It’s local to that function’s scope.
2. An instance variable is defined within a class, but outside of a class method. It’s local to the object (an instance of a class).
3. A static variable is a non-local variable that can be shared among all instances of a class (objects).
In this tutorial, to keep things simple, we will cover local variables inside the ‘main()’ function.
We won’t cover functions in this tutorial. For the moment you don’t have to worry about the ‘main’ function or the class it’s in.
To declare a variable, we specify its data type, followed by a name and a semicolon to terminate the statement.
The data type simply tells the compiler what type of value will be stored in the variable.
We cover all the data types available in Java in the lesson on Data Types .
In the example above, we declare a variable with the name ‘num’ that can store integers (whole numbers).
Our variable doesn’t have any data inside it yet, we just reserved space in memory for it.
To assign data to a variable, we specify the name of the variable, then the assignment operator = , followed by a value that matches the data type.
We only use the data type when we’re declaring or initializing the variable, not when assigning or changing a value.
In the example above, we assign the value of 5 to the variable ‘num’.
The statement reads: variable 'num' has value of 5
We will often know the value we want to assign to the variable beforehand, so we can use initialization syntax to declare a variable and assign a value to it at the same time.
To do this we simply combine the declaration and assignment together.
In the example above, we initialize a new variable with the value of 5.
We can declare or initialize multiple variables of the same type at the same time in Java.
To do this we separate each variable name with a comma. If we initialize them, we assign the value after each variable name.
Note that the elipses (…) only indicate that more variables can be declared/initialized this way. It’s not included in the syntax.
In the example above, we initialize three integer variables with separate values inline.
To use a variable, we simply specify its name wherever we need to use it in our code.
In the example above, we add the values of the ‘num1’ and ‘num2’ variables together, and store them in another variable called ‘result’.
Then we use ‘result’ in the println() function to print it to the console.
Variables in Java are mutable, which means we can change their values while the program is running (at runtime).
To change the value of a variable, we simply assign it a new one.
In the example above, we mutate the ‘result’ variable’s value in the second arithmetic and print it to the console. Then, we mutate it again by manually assigning a new value to it.
We cannot mutate a variable with just any other type of value than it was declared with.
For example, we can’t assign a string value to variable above, it would raise (generate) an error.
When we try to run the example above, the compiler raises a ‘mismatch type’ error.
The compiler couldn’t typecast the string into a number. We can only convert certain types into certain other types.
We discuss typecasting in the lesson on Data Types .
A constant is a variable that cannot have it’s value changed during runtime.
We use constants when we do not want a value to accidentally change, like the value of Pi, or gravity.
A constant cannot be declared without a value, because a value cannot be assigned later on. There would also be no point to an empty constant.
To initialize a constant with a value, we use the final keyword in front of the data type.
As mentioned in the Basic Syntax lesson , the convention for naming constants is uppercase words with underscores as word separators (uppercase snake).
In the example above, we specify that ‘PI’ is final , it cannot have its value changed at runtime.
If we try to mutate the constant, the compiler will raise an error.
Java has two types of expressions, lvalues and rvalues.
The operand on the left side of the assignment operator ( = ) is the Lvalue, and the operand on the right side is the Rvalue.
An Lvalue can have a value assigned to it so it’s allowed to appear either on the left or the right side of an assignment.
An rvalue cannot have a value assigned to it so it may only appear on the right side of an assignment.
The following quick example shows some popular types of variables we can create.
- Once the data isn’t used anymore, or the program quits, they are destroyed.
- Only that type of value is allowed to be assigned unless typecasted.
- To declare/initialize multiple variables of the same type, we separate them with a comma.
- A constant can only be initialized, and uses the final keyword in front of the type.
- Values stored in variables are mutable.
- Values stored in contants are immutable.
- To use a variable or constant, we refer to its name wherever we need to in our code.
- Variable types
- Declare a variable
- Assign a value to a variable
- Initialize a variable
- Declare multiple variables
- Use a variable
- Change a variable
- How to initialize a constant
- Operands: lvalues & rvalues
- Common variable data types

1.7 Java | Assignment Statements & Expressions
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java.
After a variable is declared, you can assign a value to it by using an assignment statement . In Java, the equal sign = is used as the assignment operator . The syntax for assignment statements is as follows:
An expression represents a computation involving values, variables, and operators that, when taking them together, evaluates to a value. For example, consider the following code:
You can use a variable in an expression. A variable can also be used on both sides of the = Â operator. For example:
In the above assignment statement, the result of x + 1 Â is assigned to the variable x . Let’s say that x is 1 before the statement is executed, and so becomes 2 after the statement execution.
To assign a value to a variable, you must place the variable name to the left of the assignment operator. Thus the following statement is wrong:
Note that the math equation x = 2 * x + 1  ≠the Java expression x = 2 * x + 1
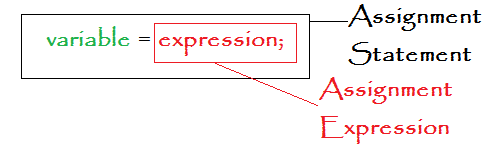
Which is equivalent to:
And this statement
is equivalent to:
Note: The data type of a variable on the left must be compatible with the data type of a value on the right. For example, int x = 1.0 would be illegal, because the data type of x is int (integer) and does not accept the double value 1.0 without Type Casting .
◄◄◄BACK | NEXT►►►
What's Your Opinion? Cancel reply
Enhance your Brain
Subscribe to Receive Free Bio Hacking, Nootropic, and Health Information
HTML for Simple Website Customization My Personal Web Customization Personal Insights
DISCLAIMER | Sitemap | â—˜
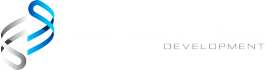
HTML for Simple Website Customization My Personal Web Customization Personal Insights SEO Checklist Publishing Checklist My Tools
Top Posts & Pages

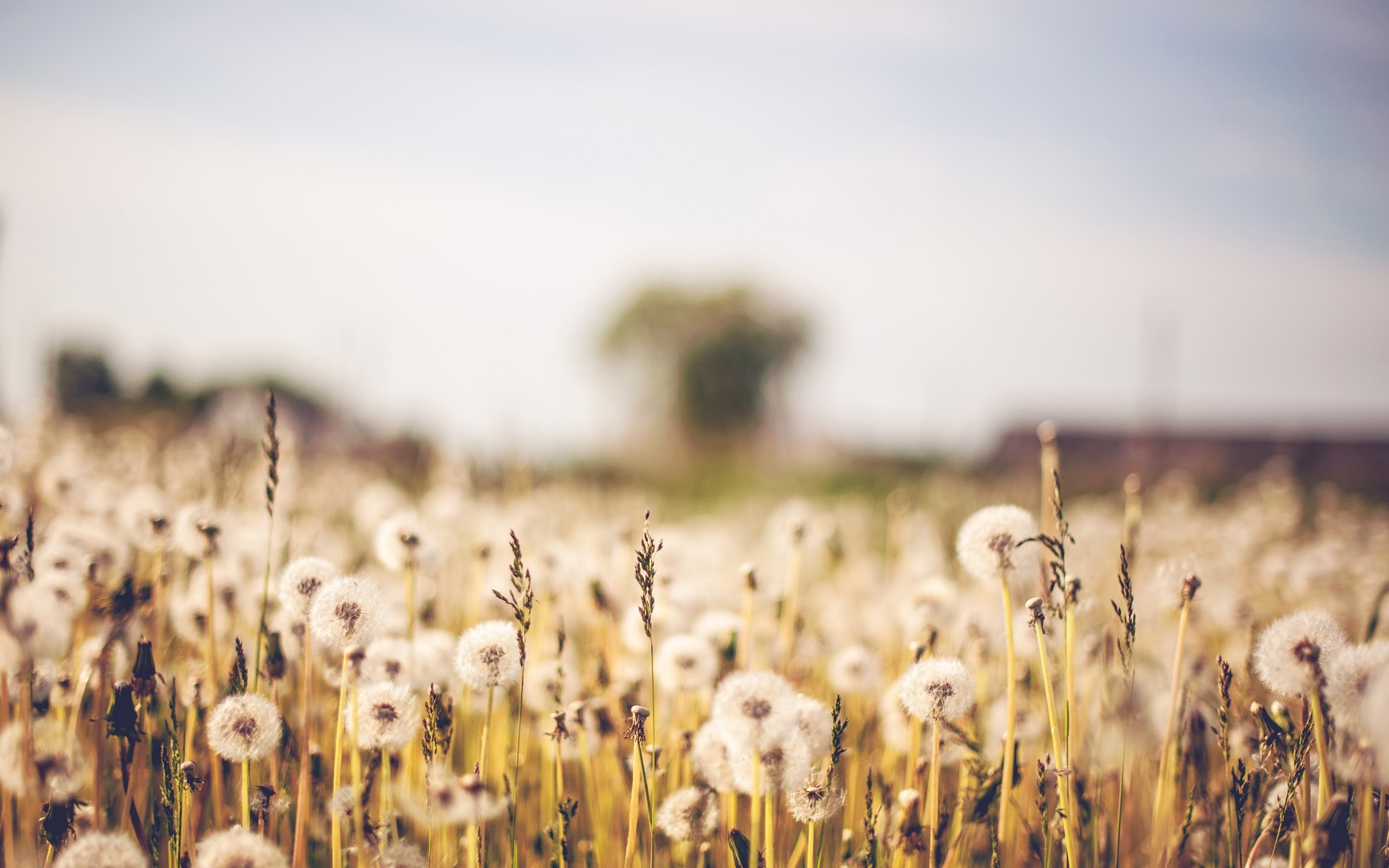
Java by examples
- Hibernate Assist
- Right Sidebar
- Left Sidebar
How to assign same value to multiple variables in Java?
Vicky thakor, 0 comments :, social media.
Interesting Posts

- Java Language Details
- For-Each Loop
- Loops and the Do-While Loop
- Switch Statement
- Jumping around - break, continue, return
Java Assignment Shortcuts
- More on Data Types
- Type Conversion and Casting
There are shortcuts in Java that let you type a little less code without introducing any new control structures.
Declaring and Assigning Variables You can declare multiple variables of the same type in one line of code:
You can also assign multiple variables to one value:
This code will set c to 5 and then set b to the value of c and finally a to the value of b .
Changing a variable One of the most common operations in Java is to assign a new value to a variable based on its current value. For example:
Since this is so common, Java let's you shorten it with a combined += operator that lets you skip the variable repetition:
(Note: Do not confuse this with index =+ 1; which would just assign positive 1 to index .)
In fact, any of the arithmetic operators can be used in this way:
j started at 3 and ended up at 5 after the above operations.
End of Free Content Preview. Please Sign in or Sign up to buy premium content.
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java variables.
Variables are containers for storing data values.
In Java, there are different types of variables, for example:
- String - stores text, such as "Hello". String values are surrounded by double quotes
- int - stores integers (whole numbers), without decimals, such as 123 or -123
- float - stores floating point numbers, with decimals, such as 19.99 or -19.99
- char - stores single characters, such as 'a' or 'B'. Char values are surrounded by single quotes
- boolean - stores values with two states: true or false
Declaring (Creating) Variables
To create a variable, you must specify the type and assign it a value:
Where type is one of Java's types (such as int or String ), and variableName is the name of the variable (such as x or name ). The equal sign is used to assign values to the variable.
To create a variable that should store text, look at the following example:
Create a variable called name of type String and assign it the value " John ":
Try it Yourself »
To create a variable that should store a number, look at the following example:
Create a variable called myNum of type int and assign it the value 15 :
You can also declare a variable without assigning the value, and assign the value later:
Note that if you assign a new value to an existing variable, it will overwrite the previous value:
Change the value of myNum from 15 to 20 :
Final Variables
If you don't want others (or yourself) to overwrite existing values, use the final keyword (this will declare the variable as "final" or "constant", which means unchangeable and read-only):
Other Types
A demonstration of how to declare variables of other types:
You will learn more about data types in the next section.
Test Yourself With Exercises
Create a variable named carName and assign the value Volvo to it.
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Array Variable Assignment in Java
- Iterating over Arrays in Java
- Array vs ArrayList in Java
- String Arrays in Java
- Array Literals in Java
- Arrays in Java
- Interesting facts about Array assignment in Java
- Java Assignment Operators with Examples
- Java Variables
- How to Create Array of Objects in Java?
- Array set() method in Java
- How to Initialize an Array in Java?
- Array Declarations in Java (Single and Multidimensional)
- How to Take Array Input From User in Java
- How Objects Can an ArrayList Hold in Java?
- Java Program to Print the Elements of an Array
- Java Array Exercise
- Array setInt() method in Java
- How to Fill (initialize at once) an Array in Java?
- Array setShort() method in Java
- Spring Boot - Start/Stop a Kafka Listener Dynamically
- Parse Nested User-Defined Functions using Spring Expression Language (SpEL)
- Split() String method in Java with examples
- Arrays.sort() in Java with examples
- For-each loop in Java
- Object Oriented Programming (OOPs) Concept in Java
- Reverse a string in Java
- HashMap in Java
- How to iterate any Map in Java
An array is a collection of similar types of data in a contiguous location in memory. After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition.
1. Element Level Promotion
Element-level promotions are not applicable at the array level. Like a character can be promoted to integer but a character array type cannot be promoted to int type array.
2. For Object Type Array
In the case of object-type arrays, child-type array variables can be assigned to parent-type array variables. That means after creating a parent-type array object we can assign a child array in this parent array.
When we assign one array to another array internally, the internal element or value won’t be copied, only the reference variable will be assigned hence sizes are not important but the type must be matched.
3. Dimension Matching
When we assign one array to another array in java, the dimension must be matched which means if one array is in a single dimension then another array must be in a single dimension. Samely if one array is in two dimensions another array must be in two dimensions. So, when we perform array assignment size is not important but dimension and type must be matched.
Please Login to comment...
Similar reads.
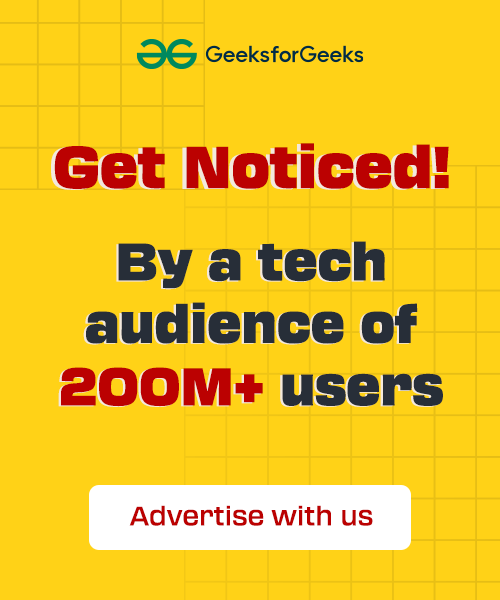
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
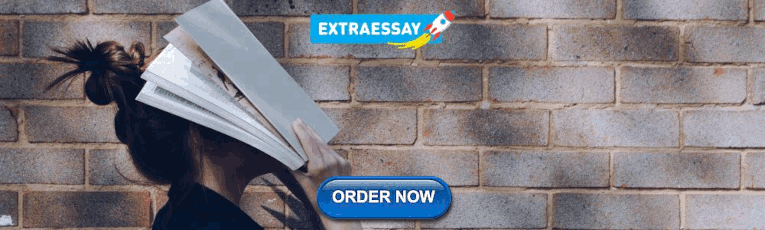
IMAGES
VIDEO
COMMENTS
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
76. You can declare multiple variables, and initialize multiple variables, but not both at the same time: String one,two,three; one = two = three = ""; However, this kind of thing (especially the multiple assignments) would be frowned upon by most Java developers, who would consider it the opposite of "visually simple".
Let's analyze the code to comprehend the intricacies of the chained assignments method. In the initial step, we declare and assign values to three integer variables (a, b, and c) in a single line, employing chained assignments.The process flows from right to left, with the rightmost value (15) assigned to c, followed by the value of c assigned to b, and ultimately, the value of b assigned to a.
In Java, you can initialize multiple variables to the same value in a single statement by separating the variables with a comma. Here is an example of how you can do this: This code initializes three int variables, x, y, and z, to the value 10. You can also use this syntax to initialize variables of different data types to the same value.
Java Declare Multiple Variables - This article is about Java Declare Multiple Variables, describing how you can declare multiple variables in one line and assign one value to multiple variables.
Multiple Assignment. I haven't said much about it, but it is legal in Java to make more than one assignment to the same variable. The effect of the second assignment is to replace the old value of the variable with a new value. int fred = 5; System.out.print (fred); fred = 7; System.out.println (fred); The output of this program is 57, because ...
Declaring Multiple Variables in Java. Fig 1: Declaring two variables on one line and initializing them together on a second line. In Java, we can use a comma-separated list to simultaneously declare multiple variables of the same type. This can make it easy to declare sets of variables, reduces the size of our code and simplifies it.
Variable types in Java. There are three types of variables in java: 1. A local variable is defined within a function, or method. It's local to that function's scope. 2. An instance variable is defined within a class, but outside of a class method. It's local to the object (an instance of a class). 3.
Java does not allow returning multiple values. Python allows this: def foo(): return 1, 2, 3. a, b, c = foo() The main point, why this does not work in Java is, that the left hand side (LHS) of the assignment must be one variable: Wrapper wrapper = WrapperGenrator.generateWrapper(); You can not assign to a tuple on the LHS as you can in Python.
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java. After a variable is declared, you can assign a value to it by using an assignment statement. ... //If a value is assigned to multiple variables, you can use this syntax: i = j = k = 1; is equivalent to: k = 1; j = k; i = j; ...
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
Following excerpt shows assignment of same value to multiple variables in Java. Source code /** * Example: Assign same value to multiple variable.
Java Assignment Shortcuts. There are shortcuts in Java that let you type a little less code without introducing any new control structures. You can also assign multiple variables to one value: This code will set c to 5 and then set b to the value of c and finally a to the value of b. One of the most common operations in Java is to assign a new ...
9. Python's multiple assignment is fairly powerful in that it can also be used for parallel assignment, like this: (x,y) = (y,x) # Swap x and y. There is no equivalent for parallel assignment in Java; you'd have to use a temporary variable: t = x; x = y; y = t; You can assign several variables from expressions in a single line like this:
Explanation: Options (a) and (b) are incorrect statements. You can define multiple variables of the same type on the same line. Also, you can assign values to variables of compatible types on the same line of code. Assignment starts from right to left. For proof, the following lines of code will compile:
This video is about a Java Puzzler - How to fix Multiple Assignments To The Same Variable in a Single ExpressionCheckout the Playlists: 👉 Java Tutorial For ...
In Java, there are different types of variables, for example: String - stores text, such as "Hello". String values are surrounded by double quotes. int - stores integers (whole numbers), without decimals, such as 123 or -123. float - stores floating point numbers, with decimals, such as 19.99 or -19.99. char - stores single characters, such as ...
After Declaring an array we create and assign it a value or variable. During the assignment variable of the array things, we have to remember and have to check the below condition. 1. Element Level Promotion. Element-level promotions are not applicable at the array level. Like a character can be promoted to integer but a character array type ...
Can't leave a comment, but want to say that what @ernest_k posted assigning multiple variables on one line in the comment above is a good catch. My code is much cleaner now, thanks! ... Multiple assignment at once in java. 0. Assigning values to an array. 2. Java: instanciate variables from array. 9.
To read multiple inputs on the same line, we can use the Scanner class along with the next () or nextLine () method. However, it would help if we used a delimiter to separate each input. 2.1. Using Space as Delimiter. One way to read multiple inputs on the same line is to use a space as a delimiter. Here's an example:
How do I assign values to multiple varibles from one line of string. For example. String time = sc.next(); // the amount of time to delay from shutdown String timeframe = sc.next(); // time frame : ... Assigning 2 string values into one variable in Java. 0. How to iterate multiple String input in Java using the Scanner class.